You can use the Toast tender API to integrate your tender provider with the Toast POS system. The tender API supports the following:
-
Scan-to-Pay Payments: Enables a guest to pay at the Toast POS system by scanning a QR code.
-
Hotel Property Management System Integration: Enables a hotel restaurant to directly bill a guest at the hotel, for example, by using their room number or name.
Additionally, the API lets you apply discounts originated from a third-party system and refund the payment and discount to a check for these features.
At a high level, the API is defined by:
-
Transaction requests
-
Transaction responses
-
Error codes
Transaction types define the action to take and related payload data. These transactions between the Toast POS system and the tender provider during a tender transaction are represented in the following figure.
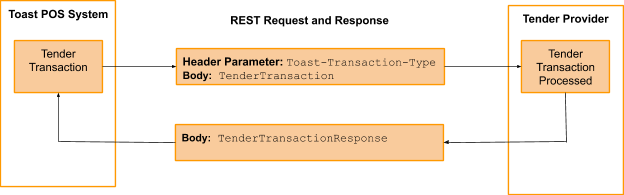
Your implementation is responsible for returning the response data synchronously.
Tender integrations define a single HTTPS endpoint. In your implementation, all requests are posted to this single endpoint. Requests from all restaurants configured to use your service will be sent to your endpoint. For example, you can name your endpoint to be:
https://<yourendpoint>.com
Each POST
request to the endpoint includes:
-
A
TenderTransaction
object in the message body. -
The following header parameters:
Header Parameter
Description
Toast-Transaction-Type
The type of tender transaction that occurred. Requests are discriminated based upon this parameter.
Toast-Restaurant-External-ID
The unique identifier of the restaurant, defined by the Toast POS system. This parameter determines which restaurant is sending the request.
Toast-Transaction-GUID
A unique identifier of the tender transaction, defined by the Toast POS system.
Authorization
A JSON Web Token (JWT) used to authenticate the request. This API key is provided by the tender partner and can be found in the request header. For more information, see Authenticating outbound API requests.
For more information about the transaction descriptions, workflows and examples, see:
-
Transaction Descriptions for scan-to-pay payments
-
Transaction Descriptions for hotel property management systems integration
The scan-to-pay payments feature allows guests and restaurant employees to scan a QR code for payment. The following sections provide more information about building a scan-to-pay payments integration for the Toast POS system:
The tender API supports the following transaction types for the scan to pay workflow:
-
TENDER_RETRIEVE_DISCOUNTS
- Request to retrieve applicable discounts for a check. -
TENDER_RETRIEVE_PAYMENTS
- Request to retrieve payment for a check. -
TENDER_REDEEM
- Confirm payment and discounts applied to a check. -
TENDER_GRATUITY
- Add a tip to a previously confirmed payment. -
TENDER_REVERSE
- Reverse a previous redemption or gratuity.
The following sections provide more information about the Toast tender transaction types:
Retrieving discounts is the first transaction that occurs when a guest or restaurant employee uses tender as the form of payment on the Toast POS system.
Retrieve Discounts Workflow
-
Guest or restaurant employee clicks Pay to pay the check.
-
Guest or restaurant employee selects the tender payment type.
-
Guest or restaurant employee scans the QR code.
-
The Toast POS system sends a
TENDER_RETRIEVE_DISCOUNTS
request to the tender provider. The request includes aTenderTransaction
object that holds the scanned QR code (tenderIdentifier
) and check (Check
) in adiscountsTransactionInformation
object. -
Your tender integration figures out the discounts to be applied to the check and responds to the Toast POS system with a
discountsResponse
value in theTenderTransactionResponse
object, which contains discount information. Note that the number of discounts can be zero. -
The Toast POS system applies the discounts and adds or updates the
appliedDiscounts
value of theCheck
object.
Retrieve Discounts Request Body
{ "discountsTransactionInformation": { "tenderIdentifier": "2670f8d0-c9c1-4dd1-b234-6922a81a7792", "orderGuid": "04ade72f-28c9-441c-a197-16d42c4c8f84", "check":{ "guid":"5689b595-e4d8-4604-a890-450b1bb86b39", "entityType":null, ... } "totalDiscountable":10.99 }, "paymentsTransactionInformation":null, "redeemTransactionInformation":null, "gratuityTransactionInformation":null, "reverseTransactionInformation":null }
Successful Retrieve Discounts Response
{ "discountsResponse":{ "account":{ "tenderIdentifier":"2670f8d0-c9c1-4dd1-b234-6922a81a7792", "properties":[ { "key":"name", "value":"james smith" } ] }, "tenderDiscounts":[ { "name":"Tender Discount", "identifier":"31d6cdf2-e766-4754-8759-f8a0f17aa9cf", "amount":5.00, "selectionGuid":"145071fe-ef70-4dda-a9ce-520bde54abca" }, { "name":"Tender Discount", "identifier":"0e557a20-b36d-4be4-9367-221d3d082780", "amount":4.00 } ] }, "transactionStatus":"ACCEPT" }
The retrieved discounts tenderDiscounts
can
be check-level or item-level discounts.
The Toast POS system retrieves payments after applying the discounts received from the tender provider.
Retrieve Payments Workflow
-
The Toast POS system sends a
TENDER_RETRIEVE_PAYMENTS
request to the tender provider. The request includes aTenderTransaction
object that holds the scanned QR code (tenderIdentifier
) and the updatedCheck
object in apaymentsTransactionInformation
object. -
Your tender integration figures out the payment and responds to the Toast POS system with a
paymentsResponse
value in theTenderTransactionResponse
object, which includes the payment information. -
The Toast POS system applies the payment and updates the
Check
object.
Retrieve Payments Request Body
{ "discountsTransactionInformation":null, "paymentsTransactionInformation": { "tenderIdentifier": "2670f8d0-c9c1-4dd1-b234-6922a81a7792", "amount": 2.11, "tipAmount":0.00, "orderGuid": "04ade72f-28c9-441c-a197-16d42c4c8f84", "check":{ ... "appliedDiscounts":[ { "guid":"26595a7b-9ad9-497a-aa45-4539989e10a2", "entityType":"AppliedExternalDiscount", "externalId":null, "approver":null, "processingState":"PENDING_APPLIED", "loyaltyDetails":null, "name":"Tender Discount", "comboItems":[ ], "discountAmount":4.0, "discount":null, "nonTaxDiscountAmount":null, "triggers":[ ], "appliedPromoCode":null } ], ... "selections":[ ... "appliedDiscounts":[ { "guid":"6b11b9d2-7a3e-4b52-9c74-b451e41ec306", "entityType":"AppliedExternalDiscount", "externalId":null, "approver":null, "processingState":"PENDING_APPLIED", "loyaltyDetails":null, "name":"Tender Discount", "comboItems":[ ], "discountAmount":5.0, "discount":null, "nonTaxDiscountAmount":null, "triggers":[ ], "appliedPromoCode":null } ], ... }, "tenderDiscountsApplied":[ { "name":"Tender Discount", "identifier":"0e557a20-b36d-4be4-9367-221d3d082780", "amount":4.00, "selectionGuid":null, "appliedDiscountGuid":"26595a7b-9ad9-497a-aa45-4539989e10a2" }, { "name":"Tender Discount", "identifier":"31d6cdf2-e766-4754-8759-f8a0f17aa9cf", "amount":5.00, "selectionGuid":"145071fe-ef70-4dda-a9ce-520bde54abca", "appliedDiscountGuid":"6b11b9d2-7a3e-4b52-9c74-b451e41ec306" } ] }, "redeemTransactionInformation":null, "gratuityTransactionInformation":null, "reverseTransactionInformation":null }
Successful Retrieve Payments Response
{ "paymentsResponse":{ "account":{ "tenderIdentifier":"2670f8d0-c9c1-4dd1-b234-6922a81a7792", "properties":[ { "key":"name", "value":"james smith" } ] }, "tenderPayments":[ { "name":"Tender Payment", "identifier":"b1727f60-a5ce-4391-9ed6-e37e8a92f1b9", "type":"STORED_VALUE", "amount":2.11, "tipAmount":0 } ] }, "transactionStatus":"ACCEPT" }
After the Toast POS system applies payments to the check, the discounts and payments are redeemed.
Redeem Discounts and Payments Workflow
-
The Toast POS system sends a
TENDER_REDEEM
request to the tender provider -
The tender provider marks the discount as used and approves the payment.
-
The restaurant employee closes the check.
Redeem Discounts and Payments Request Body
{ "discountsTransactionInformation":null, "paymentsTransactionInformation":null, "redeemTransactionInformation": { "tenderIdentifier": "2670f8d0-c9c1-4dd1-b234-6922a81a7792", "orderGuid": "04ade72f-28c9-441c-a197-16d42c4c8f84", "check":{ ... "payments":[ { "guid":"af10f95f-9ee7-4975-933d-afa2cf6806e2", "entityType":null, "externalId":null, "originalProcessingFee":null, "amount":2.11, "tipAmount":0.0, "amountTendered":0.0, "cashDrawer":null, "cardType":null, "lastModifiedDevice":null, "refundStatus":"NONE", "houseAccount":null, "type":"OTHER", "voidInfo":null, "otherPayment":{ "guid":"8cf15698-6683-4620-93e0-faf95d03273c", "entityType":"AlternatePaymentType", "externalId":"100000000100011624" }, "mcaRepaymentAmount":null, "createdDevice":null, "paidDate":"2019-12-02T15:28:02.800+0000", "cardEntryMode":null, "cardPaymentId":null, "paymentStatus":"PROCESSING", "paidBusinessDate":20191202, "last4Digits":null, "refund":null } ... }, "tenderPaymentsApplied": [ { "name": "Tender Payment", "identifier": "b1727f60-a5ce-4391-9ed6-e37e8a92f1b9", "type": "STORED_VALUE", "amount": 2.11, "tipAmount": 0.0, "paymentGuid":"af10f95f-9ee7-4975-933d-afa2cf6806e2" } ], "tenderDiscountsApplied": [ { "name": "Tender Discount", "identifier": "0e557a20-b36d-4be4-9367-221d3d082780", "amount": 4.0, "selectionGuid":null, "appliedDiscountGuid":"26595a7b-9ad9-497a-aa45-4539989e10a2" }, { "name":"Tender Discount", "identifier":"31d6cdf2-e766-4754-8759-f8a0f17aa9cf", "amount":5.00, "selectionGuid":"145071fe-ef70-4dda-a9ce-520bde54abca", "appliedDiscountGuid":"6b11b9d2-7a3e-4b52-9c74-b451e41ec306" } ] }, "gratuityTransactionInformation":null, "reverseTransactionInformation":null }
After the Toast POS system receives a response from the tender provider about the discounts and payments redemption, it prompts the restaurant guest for a gratuity.
Gratuity Workflow
-
In the Toast POS system, a user interface pops up and the guest chooses to add a tip. The Toast POS system sends a
TENDER_GRATUITY
request that specifies an additional gratuity amount to add to aTENDER_REDEEM
transaction. TheToast-Transaction-GUID
ofTENDER_REDEEM
to add the gratuity amount is provided in thetransactionToUpdate
value in the body of theTENDER_GRATUITY
request.Note
For some tender providers, the gratuity functionality can be disabled, because the functionality is built into the provider's app. This is configurable through Toast Web.
-
The tender provider responds to the Toast POS system with a
gratuityResponse
value in theTransactionResponseGratuity
object, which contains the value of gratuity that the guest added.
Gratuity Request Body
{ "discountsTransactionInformation":null, "paymentsTransactionInformation":null, "redeemTransactionInformation":null, "gratuityTransactionInformation":{ "transactionToUpdate":"73885a84-59c3-44b6-a4c7-45ea23892c56", "additionalGratuity":3.00 }, "reverseTransactionInformation":null }
Successful Gratuity Response Body
{ "gratuityResponse": { "account": { "tenderIdentifier": "2670f8d0-c9c1-4dd1-b234-6922a81a7792", "properties": [ ... ] }, "tenderPayments": [ { "name":"Tender Payment", "identifier":"66b74964-3aba-41c4-ad65-718c59083011", "type":"STORED_VALUE", "amount":2.11, "tipAmount":3 } ] }, "transactionStatus": "ACCEPT" }
A transaction is reversed in the following situations:
-
If the restaurant employee voids the payment, the Toast POS system sends a
TENDER_REVERSE
request to the PMS provider to reverse the payment and any applied discounts. -
If the restaurant employee voids the order, then the Toast POS system sends a
TENDER_REVERSE
request to the tender provider. -
If a discount times out during redemption, then the Toast POS system sends a
TENDER_REVERSE
request to the tender provider.
Reverse requests can be one or more of the following:
-
Payments
-
Discounts
In the request body, the value of
transactionToUpdate
is the
Toast-Transaction-GUID
of the
TENDER_REDEEM
request.
discountsToRemove
and
paymentsToRemove
contain the discounts and payment
identifiers, respectively.
Reverse Request Body
{ "discountsTransactionInformation":null, "paymentsTransactionInformation":null, "redeemTransactionInformation":null, "gratuityTransactionInformation":null, "reverseTransactionInformation": { "transactionToUpdate": "5ff4ad7f-69db-4cb1-bf25-ff250c8dc261", "discountsToRemove": [ "0e557a20-b36d-4be4-9367-221d3d082780", "31d6cdf2-e766-4754-8759-f8a0f17aa9cf" }, "paymentsToRemove": [ "b1727f60-a5ce-4391-9ed6-e37e8a92f1b9" ] } }
This section describes the user interface workflow for scan to pay tender payments in the Toast POS system.
After completing an order, a guest can fully or partially pay for the check using tender payment:
-
The restaurant employee clicks the Pay button to navigate to the Payments screen.
-
The restaurant employee clicks the Other button.
The
screen appears and the tender providers names are listed as an option. -
After selecting the tender provider name, the camera or external scanner turns on to scan the QR code.
-
After the QR code is scanned and the guest is identified, the API returns whether the transaction has been approved or has failed. Additionally, the API also ensures that any check-level or item-level discounts that are linked with the guest's account are applied to the check.
If the transaction fails, an error message appears, confirming that the ID is not valid, and the guest is returned to the Payments screen to use another payment type.
-
If the restaurant employee chose to print a receipt, your name is printed on the receipt, as shown in the snapshot below.
If discounts are applied, the name of the discount you return is also included. The following figure shows a receipt with a check-level discount applied and the remainder paid for through the tender provider app.
Property management systems (PMS) are used by hotels to coordinate systems used for servicing guests and charges. You can use the tender API to implement an integration between a PMS and the Toast POS system. The integration allows an employee at a hotel restaurant to look up a guest's account and the guest can pay a check directly to that account. The following sections provide more information about building a PMS integration for the Toast POS system:
The tender API supports the following transaction types for the PMS integration workflow:
-
TENDER_SEARCH_CONFIG
- Request configured search terms for the restaurant. -
TENDER_SEARCH
- Search for an account. -
TENDER_RETRIEVE_DISCOUNTS
- Retrieve applicable discounts for a check. -
TENDER_RETRIEVE_PAYMENTS
- Retrieve payment for a check. -
TENDER_REDEEM
- Confirm payment and discounts applied to a check. -
TENDER_GRATUITY
- Add a tip to a previously confirmed payment. -
TENDER_REVERSE
- Reverse a previous redemption or gratuity.
The following sections provide more information about the transaction types:
Configuring the search is the first transaction that occurs when a restaurant employee at a hotel restaurant initiates a workflow to charge a check to a guest's account.
Configure Search Workflow
-
A guest wants to charge a check to a hotel room.
-
A restaurant employee clicks Pay to pay the check and selects hotel room charge as the payment method on the Toast POS device.
-
The Toast POS system sends a
TENDER_SEARCH_CONFIG
request to the hotel PMS provider to get the search terms used by the provider to look up guest information. -
The PMS provider sends a response in a
searchConfigResponse
object. Each key-value pair in this object defines an input field (key
) with its type (value
).The type must be one of the following:
-
NUMBER
-
TEXT
-
EMAIL
-
PHONE_NUMBER
For example, the guest's room number or reservation number is a
NUMBER
and their name or company's name is aTEXT
.The first four search terms are displayed on the guest lookup screen on the POS device. The type of the input field also determines the type of virtual keyboard that is displayed for that input field to enter the search term.
-
The following example shows the search terms configured as room number, name, reservation number and phone number.
Configure Search Request
The TENDER_SEARCH_CONFIG
transaction is a
POST
request to the API endpoint
https://api.<yourendpoint>.com
. It has the
following header parameters:
-
Toast-Transaction-Type
-
Toast-Restaurant-External-ID
-
Toast-Transaction-GUID
-
Authorization
This request has no body.
Successful Configure Search Response
{ "searchConfigResponse": { "searchTermNames": [ { "key": "Room Number", "value": "NUMBER" }, { "key": "Name", "value": "TEXT" }, { "key": "Reservation Number", "value": "NUMBER" } { "key": "Phone Number", "value": "PHONE_NUMBER" } ] }, "transactionStatus": "ACCEPT" }
After a search configuration request transaction, a Toast POS device displays the guest search criteria. A restaurant employee can search for a guest on the Toast POS device using a guest's information, such as name, room number or account number.
Search Workflow
-
A restaurant employee enters the guest's identifying information in the input fields on the Toast POS device and searches for the guest.
-
The Toast POS device sends a
TENDER_SEARCH
request to the PMS provider. The request is aTenderTransaction
object that contains asearchTransactionInformation
object which contains asearchTerms
object. ThissearchTerms
object contains the search criteria, in the form of a key/value pair:-
The
key
corresponds to the input field where the restaurant employee enters the guest's identifying information. -
The
value
corresponds to the string that the employee types on the Toast POS device. Thevalue
can be partial such as only first name or last name or partial name.
-
-
The PMS provider returns the results of the search request in a
searchResponse
object that contains asearchResults
property. ThesearchResults
property contains an array ofAccountInfo
objects. Each entry in the array corresponds to a guest and has:-
An identifier for the guest (
tenderIdentifier
). -
The search results returned as key-value pairs with the guest information such as name and room number. The search results are displayed on the Toast POS device.
-
The following example shows a search for a guest whose name
contains john
. The PMS provider responds with two
results for john adams
and tommy
john
with their information.
Search Request Body
{ "searchTransactionInformation": { "searchTerms": [ { "key": "Name", "value": "john" } ] } }
Successful Search Response
{ "searchResponse": { "searchResults": [ { "tenderIdentifier": "2670f8d0-c9c1-4dd1-b234-6922a81a7792", "properties": [ { "key": "room number", "value": "809" }, { "key": "name", "value": "john adams" }, { "key": "reservation number", "value": "12531953" }, { "key": "email", "value": "a2@example.com" }, { "key": "phone number", "value": null } ] }, { "tenderIdentifier": "4670f8d0-c9c1-4dd1-b234-6922a81a7792", "properties": [ { "key": "room number", "value": "1234" }, { "key": "name", "value": "tommy john" }, { "key": "reservation number", "value": "13623005" }, { "key": "email", "value": "a3@example.com" }, { "key": "phone number", "value": null } ] } ] }, "transactionStatus": "ACCEPT" }
After a restaurant employee selects a guest from the search results on the Toast POS device, the Toast POS system retrieves discounts from the PMS provider.
Retrieve Discounts Workflow
-
The Toast POS system sends a
TENDER_RETRIEVE_DISCOUNTS
request to the PMS provider. The request includes aTenderTransaction
object that holds the guest identifier (tenderIdentifier
) and check (Check
) in adiscountsTransactionInformation
object. -
Your PMS integration figures out the discounts to be applied to the check and responds to the Toast POS system with a
discountsResponse
value in theTenderTransactionResponse
object, which contains discount information. Note that the number of discounts can be zero. -
The Toast POS system applies the discounts and adds or updates the
appliedDiscounts
value of theCheck
object.
Retrieve Discounts Request Body
{ "discountsTransactionInformation": { "tenderIdentifier": "2670f8d0-c9c1-4dd1-b234-6922a81a7792", "orderGuid": "04ade72f-28c9-441c-a197-16d42c4c8f84", "check":{ "guid":"5689b595-e4d8-4604-a890-450b1bb86b39", "entityType":null, ... } "totalDiscountable":10.99 }, "paymentsTransactionInformation":null, "redeemTransactionInformation":null, "gratuityTransactionInformation":null, "reverseTransactionInformation":null }
Successful Retrieve Discounts Response
{ "discountsResponse":{ "account":{ "tenderIdentifier":"2670f8d0-c9c1-4dd1-b234-6922a81a7792", "properties":[ { "key":"name", "value":"john adams" } ] }, "tenderDiscounts":[ { "name":"Tender Discount", "identifier":"31d6cdf2-e766-4754-8759-f8a0f17aa9cf", "amount":5.00, "selectionGuid":"145071fe-ef70-4dda-a9ce-520bde54abca" }, { "name":"Tender Discount", "identifier":"0e557a20-b36d-4be4-9367-221d3d082780", "amount":4.00 } ] }, "transactionStatus":"ACCEPT" }
The retrieved discounts tenderDiscounts
can
be check-level or item-level discounts.
The Toast POS system retrieves payments after applying the discounts received from the PMS provider.
Retrieve Payments Workflow
-
The Toast POS system sends a
TENDER_RETRIEVE_PAYMENTS
request to the PMS provider. The request includes aTenderTransaction
object that holds the guest identifier (tenderIdentifier
) and the updatedCheck
object in apaymentsTransactionInformation
object. -
Your tender integration figures out the payment and responds to the Toast POS system with a
paymentsResponse
value in theTenderTransactionResponse
object, which includes the payment information. -
The Toast POS system applies the payment and updates the
Check
object.
Retrieve Payments Request Body
{ "discountsTransactionInformation":null, "paymentsTransactionInformation": { "tenderIdentifier": "2670f8d0-c9c1-4dd1-b234-6922a81a7792", "amount": 2.11, "tipAmount":0.00, "orderGuid": "04ade72f-28c9-441c-a197-16d42c4c8f84", "check":{ ... "appliedDiscounts":[ { "guid":"26595a7b-9ad9-497a-aa45-4539989e10a2", "entityType":"AppliedExternalDiscount", "externalId":null, "approver":null, "processingState":"PENDING_APPLIED", "loyaltyDetails":null, "name":"Tender Discount", "comboItems":[ ], "discountAmount":4.0, "discount":null, "nonTaxDiscountAmount":null, "triggers":[ ], "appliedPromoCode":null } ], ... "selections":[ ... "appliedDiscounts":[ { "guid":"6b11b9d2-7a3e-4b52-9c74-b451e41ec306", "entityType":"AppliedExternalDiscount", "externalId":null, "approver":null, "processingState":"PENDING_APPLIED", "loyaltyDetails":null, "name":"Tender Discount", "comboItems":[ ], "discountAmount":5.0, "discount":null, "nonTaxDiscountAmount":null, "triggers":[ ], "appliedPromoCode":null } ], ... }, "tenderDiscountsApplied":[ { "name":"Tender Discount", "identifier":"0e557a20-b36d-4be4-9367-221d3d082780", "amount":4.00, "selectionGuid":null, "appliedDiscountGuid":"26595a7b-9ad9-497a-aa45-4539989e10a2" }, { "name":"Tender Discount", "identifier":"31d6cdf2-e766-4754-8759-f8a0f17aa9cf", "amount":5.00, "selectionGuid":"145071fe-ef70-4dda-a9ce-520bde54abca", "appliedDiscountGuid":"6b11b9d2-7a3e-4b52-9c74-b451e41ec306" } ] }, "redeemTransactionInformation":null, "gratuityTransactionInformation":null, "reverseTransactionInformation":null }
Successful Retrieve Payments Response
{ "paymentsResponse":{ "account":{ "tenderIdentifier":"2670f8d0-c9c1-4dd1-b234-6922a81a7792", "properties":[ { "key":"name", "value":"john adams" } ] }, "tenderPayments":[ { "name":"Tender Payment", "identifier":"b1727f60-a5ce-4391-9ed6-e37e8a92f1b9", "type":"STORED_VALUE", "amount":2.11, "tipAmount":0 } ] }, "transactionStatus":"ACCEPT" }
After the Toast POS system applies payments to the check, the discounts and payments are redeemed.
Redeem Discounts and Payments Workflow
-
The Toast POS system sends a
TENDER_REDEEM
request to the PMS provider -
The PMS provider marks the discounts as used and adds the payment to the guest's account.
-
The restaurant employee closes the check if the full amount is covered by the room charge.
Redeem Discounts and Payments Request Body
{ "discountsTransactionInformation":null, "paymentsTransactionInformation":null, "redeemTransactionInformation": { "tenderIdentifier": "2670f8d0-c9c1-4dd1-b234-6922a81a7792", "orderGuid": "04ade72f-28c9-441c-a197-16d42c4c8f84", "check":{ ... "payments":[ { "guid":"af10f95f-9ee7-4975-933d-afa2cf6806e2", "entityType":null, "externalId":null, "originalProcessingFee":null, "amount":2.11, "tipAmount":0.0, "amountTendered":0.0, "cashDrawer":null, "cardType":null, "lastModifiedDevice":null, "refundStatus":"NONE", "houseAccount":null, "type":"OTHER", "voidInfo":null, "otherPayment":{ "guid":"8cf15698-6683-4620-93e0-faf95d03273c", "entityType":"AlternatePaymentType", "externalId":"100000000100011624" }, "mcaRepaymentAmount":null, "createdDevice":null, "paidDate":"2019-12-02T15:28:02.800+0000", "cardEntryMode":null, "cardPaymentId":null, "paymentStatus":"PROCESSING", "paidBusinessDate":20191202, "last4Digits":null, "refund":null } ... }, "tenderPaymentsApplied": [ { "name": "Tender Payment", "identifier": "b1727f60-a5ce-4391-9ed6-e37e8a92f1b9", "type": "STORED_VALUE", "amount": 2.11, "tipAmount": 0.0, "paymentGuid":"af10f95f-9ee7-4975-933d-afa2cf6806e2" } ], "tenderDiscountsApplied": [ { "name": "Tender Discount", "identifier": "0e557a20-b36d-4be4-9367-221d3d082780", "amount": 4.0, "selectionGuid":null, "appliedDiscountGuid":"26595a7b-9ad9-497a-aa45-4539989e10a2" }, { "name":"Tender Discount", "identifier":"31d6cdf2-e766-4754-8759-f8a0f17aa9cf", "amount":5.00, "selectionGuid":"145071fe-ef70-4dda-a9ce-520bde54abca", "appliedDiscountGuid":"6b11b9d2-7a3e-4b52-9c74-b451e41ec306" } ] }, "gratuityTransactionInformation":null, "reverseTransactionInformation":null }
After the Toast POS system receives a response from the PMS provider about the discounts and payments redemption, if configured it prompts the restaurant employee for a gratuity.
Note |
For some PMS providers, the gratuity functionality can be disabled. This is configurable through Toast Web. |
Gratuity Workflow
-
After a restaurant employee enters a gratuity amount, the Toast POS system sends a
TENDER_GRATUITY
request that specifies a gratuity amount to add to aTENDER_REDEEM
transaction. TheToast-Transaction-GUID
ofTENDER_REDEEM
to add the gratuity amount is provided in thetransactionToUpdate
value in the body of theTENDER_GRATUITY
request. -
The PMS provider responds to the Toast POS system with a
gratuityResponse
value in theTransactionResponseGratuity
object, which contains the value of gratuity that the guest added.
Gratuity Request Body
{ "discountsTransactionInformation":null, "paymentsTransactionInformation":null, "redeemTransactionInformation":null, "gratuityTransactionInformation":{ "transactionToUpdate":"73885a84-59c3-44b6-a4c7-45ea23892c56", "additionalGratuity":3.00 }, "reverseTransactionInformation":null }
Successful Gratuity Response Body
{ "gratuityResponse": { "account": { "tenderIdentifier": "2670f8d0-c9c1-4dd1-b234-6922a81a7792", "properties": [ ... ] }, "tenderPayments": [ { "name":"Tender Payment", "identifier":"66b74964-3aba-41c4-ad65-718c59083011", "type":"STORED_VALUE", "amount":2.11, "tipAmount":3 } ] }, "transactionStatus": "ACCEPT" }
A transaction is reversed in the following situations:
-
If the restaurant employee voids the payment, the Toast POS system sends a
TENDER_REVERSE
request to the PMS provider to reverse the payment and any applied discounts. -
If the restaurant employee voids the order, then the Toast POS system sends a
TENDER_REVERSE
request to the PMS provider. -
If a discount times out during redemption, then the Toast POS system sends a
TENDER_REVERSE
request to the PMS provider.
Reverse requests can be one or more of the following:
-
Payments
-
Discounts
-
Gratuity
A gratuity can be reversed on its own if there is a network error when the gratuity request is sent. Additionally, if a gratuity is added to a payment after
TENDER_REDEEM
and that payment is reversed, then the gratuity is also reversed.
In the request body, the value of
transactionToUpdate
is the
Toast-Transaction-GUID
of the
TENDER_REDEEM
request. The
discountsToRemove
and
paymentsToRemove
contain the discounts and payment
identifiers, respectively.
Reverse Request Body
{ "discountsTransactionInformation":null, "paymentsTransactionInformation":null, "redeemTransactionInformation":null, "gratuityTransactionInformation":null, "reverseTransactionInformation": { "transactionToUpdate": "5ff4ad7f-69db-4cb1-bf25-ff250c8dc261", "discountsToRemove": [ "0e557a20-b36d-4be4-9367-221d3d082780", "31d6cdf2-e766-4754-8759-f8a0f17aa9cf" }, "paymentsToRemove": [ "b1727f60-a5ce-4391-9ed6-e37e8a92f1b9" ] } }
This section describes the user interface workflow in the Toast POS system for billing a hotel restaurant guest using the hotel's PMS provider.
At the payment phase of the Toast POS system order workflow:
-
A guest chooses to pay a balance due by applying the balance to their room charge.
-
A restaurant employee clicks the Pay button to navigate to the Payments screen.
-
The restaurant employee clicks the Other button.
The
screen appears and room charge is listed as an option. -
After selecting room charge, a Lookup Hotel Guest screen appears.
The restaurant employee can look up a guest by their room number or name or a combination of search terms.
-
The restaurant employee enters, for example, a room number in the Enter Room # field and selects Search.
-
Once the search is complete, a list of search results appears on the Toast POS device.
-
The restaurant employee selects the record corresponding to the guest that owes the check amount and processes the payment with the PMS provider. If digital receipts are configured, the Toast POS device prompts the restaurant employee to add the tip amount.
If the restaurant employee chose to print a receipt, the guest's name and discounts are printed on the receipt.
-
The restaurant employee can go back to the check on the Toast POS device and see the type of charge.
-
You cannot use the tender API to pay for checks using the orders API or Toast Online Ordering. The tender API is only supported at the Toast POS system.
-
You cannot use the tender API with contactless pay.
Your tender interface implementation must return the following HTTPS responses to the requests that it receives from the Toast POS system.
200 means OK and has pre-defined success status in the response
-
ACCEPT
- The tender service provider processed the transaction successfully.
400 means a bad request. The transactionStatus
value of the TenderTransactionResponse
object is one
of:
-
ERROR_INVALID_TOAST_TRANSACTION_TYPE
- Your implementation does not handle the transaction type that was supplied in the request. Return this response status type when the request transaction type is one of the types defined by the tender integration API specification but you have decided not to support it. -
ERROR_INVALID_INPUT_PROPERTIES
- The JSON values specified in thetenderTransaction
object in the request body do not match the requirements of the transaction type. -
ERROR_INVALID_TOKEN
- The authentication token supplied in theAuthorization
header parameter is invalid or cannot be validated. For information about request authentication, see Authenticating outbound API requests. -
ERROR_INVALID_RESTAURANT
- The restaurant identifier included in theToast-Restaurant-External-ID
header parameter of the request is not recognized by the tender provider integration implementation. You receive the identifiers for the Toast POS system restaurants from the Toast technical partnership team when you implement your tender integration. -
ERROR_TRANSACTION_DOES_NOT_EXIST
- The transaction that is being requested to be reversed, specified by aTENDER_REVERSE
request, does not exist. -
ERROR_TRANSACTION_CANNOT_BE_REVERSED
- The transaction specified by aTENDER_REVERSE
request cannot be reversed. Transactions that have already been reversed. -
ERROR_ACCOUNT_INVALID
- The tender identifier is not in the set of identifiers that correspond to the current restaurant. For example, if a guest account is entered incorrectly, your implementation returns this status. -
ERROR_INSUFFICIENT_FUNDS
- The payment or tip, specified in aTENDER_RETRIEVE_PAYMENTS
orTENDER_RETRIEVE_GRATUITY
request, cannot be retrieved because the tender account does not have sufficient balance. -
ERROR_UNABLE_TO_PROCESS
- The transaction cannot be processed by the partner. This can be due to an issue that occurred on the partner's end.
500 means Internal Server Error
The Toast POS system expects a 500ms average response time from your tender API endpoint. The maximum response time must be 5 seconds, after which we may close the socket and send another request. Response times should be as short as possible because there will be a server waiting.